Note
To get the output project and results from this example in your Python session, run:
from RATapi.examples import DSPC_standard_layers
project, results = DSPC_standard_layers()
[1]:
import pathlib
import numpy as np
import RATapi as RAT
from RATapi.models import Layer, Parameter
Standard Layers Analysis of a DSPC Floating Bilayer#
In this worksheet, we will carry out an analysis of a floating bilayer sample using a ‘standard layers’ model. The sample consists of a DSPC bilayer, on a silane SAM on Silicon:
So we are going to need layers for Oxide, SAM tails, SAM heads, and the Heads/Tails of the Bilayer. We also need to consider hydration of the submerged bilayer.
Making the Project#
Start by initialising a project:
[2]:
problem = RAT.Project(name="original_dspc_bilayer", calculation="normal", model="standard layers", geometry="substrate/liquid", absorption=False)
The add the parameters we are going to need:
[3]:
parameter_list = [
Parameter(name="Oxide Thickness", min=5.0, value=19.54, max=60.0, fit=True, prior_type="uniform"),
Parameter(name="Oxide SLD", min=3.39e-06, value=3.39e-06, max=3.41e-06, fit=False, prior_type="uniform"),
Parameter(name="Oxide Hydration", min=0.0, value=23.61, max=60.0, fit=True, prior_type="uniform"),
#
Parameter(name="SAM Tails Thickness", min=15.0, value=22.66, max=35.0, fit=True, prior_type="uniform"),
Parameter(name="SAM Tails SLD", min=-5e-07, value=-4.01e-07, max=-3e-07, fit=False, prior_type="uniform"),
Parameter(name="SAM Tails Hydration", min=1.0, value=5.252, max=50.0, fit=True, prior_type="uniform"),
Parameter(name="SAM Roughness", min=1.0, value=5.64, max=15.0, fit=True, prior_type="uniform"),
#
Parameter(name="SAM Heads Thickness", min=5.0, value=8.56, max=17.0, fit=True, prior_type="gaussian", mu=10.0, sigma=2.0),
Parameter(name="SAM Heads SLD", min=1.0e-07, value=1.75e-06, max=2.0e-06, fit=False, prior_type="uniform"),
Parameter(name="SAM Heads Hydration", min=10.0, value=45.45, max=50.0, fit=True, prior_type="gaussian", mu=30.0, sigma=3.0),
#
Parameter(name="CW Thickness", min=10.0, value=17.12, max=28.0, fit=True, prior_type="uniform"),
Parameter(name="CW SLD", min=0.0, value=0.0, max=1e-09, fit=False, prior_type="uniform"),
Parameter(name="CW Hydration", min=99.9, value=100.0, max=100.0, fit=False, prior_type="uniform"),
#
Parameter(name="Bilayer Heads Thickness", min=7.0, value=10.7, max=17.0, fit=True, prior_type="gaussian", mu=10.0, sigma=2.0),
Parameter(name="Bilayer Heads SLD", min=5.0e-07, value=1.47e-06, max=1.5e-06, fit=False, prior_type="uniform"),
Parameter(name="Bilayer Heads Hydration", min=10.0, value=36.15, max=50.0, fit=True, prior_type="gaussian", mu=30.0, sigma=3.0),
Parameter(name="Bilayer Roughness", min=2.0, value=6.014, max=15.0, fit=True, prior_type="uniform"),
Parameter(name="Bilayer Tails Thickness", min=14.0, value=17.82, max=22.0, fit=True, prior_type="uniform"),
Parameter(name="Bilayer Tails SLD", min=-5.0e-07, value=-4.61e-07, max=0.0, fit=False, prior_type="uniform"),
Parameter(name="Bilayer Tails Hydration", min=10.0, value=17.64, max=50.0, fit=True, prior_type="uniform")
]
problem.parameters.extend(parameter_list)
# In addition to these, there is also Substrate Roughness which is always parameter 1. Increase the allowed range of this a bit
problem.parameters.set_fields(0, max=10)
Now we can group these parameters into the layers we need, and add them to the project:
[4]:
layers = [
Layer(name="Oxide", thickness="Oxide Thickness", SLD="Oxide SLD", roughness="Substrate Roughness",
hydration="Oxide Hydration", hydrate_with="bulk out"),
Layer(name="SAM Tails", thickness="SAM Tails Thickness", SLD="SAM Tails SLD", roughness="SAM Roughness",
hydration="SAM Tails Hydration", hydrate_with="bulk out"),
Layer(name="SAM Heads", thickness="SAM Heads Thickness", SLD="SAM Heads SLD", roughness="SAM Roughness",
hydration="SAM Heads Hydration", hydrate_with="bulk out"),
Layer(name="Central Water", thickness="CW Thickness", SLD="CW SLD", roughness="Bilayer Roughness",
hydration="CW Hydration", hydrate_with="bulk out"),
Layer(name="Bilayer Heads", thickness="Bilayer Heads Thickness", SLD="Bilayer Heads SLD", roughness="Bilayer Roughness",
hydration="Bilayer Heads Hydration", hydrate_with="bulk out"),
Layer(name="Bilayer Tails", thickness="Bilayer Tails Thickness", SLD="Bilayer Tails SLD", roughness="Bilayer Roughness",
hydration="Bilayer Tails Hydration", hydrate_with="bulk out")
]
problem.layers.extend(layers)
Now deal with the experimental parameters. We will delete the predefined default parameters and add new ones for this specific problem. We need a bulk in of Silicon, and two Bulk outs - D2O and SMW.
[5]:
del problem.bulk_in[0]
problem.bulk_in.append(name="Silicon", min=2.0e-06, value=2.073e-06, max=2.1e-06, fit=False)
del problem.bulk_out[0]
problem.bulk_out.append(name="D2O", min=5.5e-06, value=5.98e-06, max=6.4e-06, fit=True)
problem.bulk_out.append(name="SMW", min=1.0e-06, value=2.21e-06, max=4.99e-06, fit=True)
Likewise the scalefactors and backgrounds:
[6]:
del problem.scalefactors[0]
problem.scalefactors.append(name="Scalefactor 1", min=0.05, value=0.10, max=0.2, fit=False)
problem.scalefactors.append(name="Scalefactor 2", min=0.05, value=0.15, max=0.2, fit=False)
# Now deal with the backgrounds
del problem.backgrounds[0]
del problem.background_parameters[0]
problem.background_parameters.append(name="Background parameter D2O", min=5.0e-10, value=2.23e-06, max=7.0e-06, fit=True)
problem.background_parameters.append(name="Background parameter SMW", min=1.0e-10, value=3.38e-06, max=4.99e-06, fit=True)
problem.backgrounds.append(name="D2O Background", type="constant", source="Background parameter D2O")
problem.backgrounds.append(name="SMW Background", type="constant", source="Background parameter SMW")
Now load in and add the data:
[7]:
data_path = pathlib.Path("../data")
d2o_dat = np.loadtxt(data_path / "DSPC_D2O.dat", delimiter=",")
problem.data.append(name="dspc_bil_D2O", data=d2o_dat)
smw_dat = np.loadtxt(data_path / "DSPC_SMW.dat", delimiter=",")
problem.data.append(name="dspc_bil_smw", data=smw_dat)
Finally, we build everything up into the two contrasts:
[8]:
# Set the model
stack = ["Oxide", "SAM Tails", "SAM Heads", "Central Water", "Bilayer Heads", "Bilayer Tails", "Bilayer Tails", "Bilayer Heads"]
# Then make the two contrasts
problem.contrasts.append(
name="D2O",
bulk_in="Silicon",
bulk_out="D2O",
background="D2O Background",
resolution="Resolution 1",
scalefactor="Scalefactor 1",
data="dspc_bil_D2O",
model=stack,
)
problem.contrasts.append(
name="SMW",
bulk_in="Silicon",
bulk_out="SMW",
background="SMW Background",
resolution="Resolution 1",
scalefactor="Scalefactor 2",
data="dspc_bil_smw",
model=stack,
)
Print our project, to check what we have:
[9]:
print(problem)
Name: ----------------------------------------------------------------------------------------------
original_dspc_bilayer
Calculation: ---------------------------------------------------------------------------------------
normal
Model: ---------------------------------------------------------------------------------------------
standard layers
Geometry: ------------------------------------------------------------------------------------------
substrate/liquid
Parameters: ----------------------------------------------------------------------------------------
+-------+-------------------------+----------+-----------+----------+-------+
| index | name | min | value | max | fit |
+-------+-------------------------+----------+-----------+----------+-------+
| 0 | Substrate Roughness | 1.0 | 3.0 | 10.0 | True |
| 1 | Oxide Thickness | 5.0 | 19.54 | 60.0 | True |
| 2 | Oxide SLD | 3.39e-06 | 3.39e-06 | 3.41e-06 | False |
| 3 | Oxide Hydration | 0.0 | 23.61 | 60.0 | True |
| 4 | SAM Tails Thickness | 15.0 | 22.66 | 35.0 | True |
| 5 | SAM Tails SLD | -5e-07 | -4.01e-07 | -3e-07 | False |
| 6 | SAM Tails Hydration | 1.0 | 5.252 | 50.0 | True |
| 7 | SAM Roughness | 1.0 | 5.64 | 15.0 | True |
| 8 | SAM Heads Thickness | 5.0 | 8.56 | 17.0 | True |
| 9 | SAM Heads SLD | 1e-07 | 1.75e-06 | 2e-06 | False |
| 10 | SAM Heads Hydration | 10.0 | 45.45 | 50.0 | True |
| 11 | CW Thickness | 10.0 | 17.12 | 28.0 | True |
| 12 | CW SLD | 0.0 | 0.0 | 1e-09 | False |
| 13 | CW Hydration | 99.9 | 100.0 | 100.0 | False |
| 14 | Bilayer Heads Thickness | 7.0 | 10.7 | 17.0 | True |
| 15 | Bilayer Heads SLD | 5e-07 | 1.47e-06 | 1.5e-06 | False |
| 16 | Bilayer Heads Hydration | 10.0 | 36.15 | 50.0 | True |
| 17 | Bilayer Roughness | 2.0 | 6.014 | 15.0 | True |
| 18 | Bilayer Tails Thickness | 14.0 | 17.82 | 22.0 | True |
| 19 | Bilayer Tails SLD | -5e-07 | -4.61e-07 | 0.0 | False |
| 20 | Bilayer Tails Hydration | 10.0 | 17.64 | 50.0 | True |
+-------+-------------------------+----------+-----------+----------+-------+
Bulk In: -------------------------------------------------------------------------------------------
+-------+---------+-------+-----------+---------+-------+
| index | name | min | value | max | fit |
+-------+---------+-------+-----------+---------+-------+
| 0 | Silicon | 2e-06 | 2.073e-06 | 2.1e-06 | False |
+-------+---------+-------+-----------+---------+-------+
Bulk Out: ------------------------------------------------------------------------------------------
+-------+------+---------+----------+----------+------+
| index | name | min | value | max | fit |
+-------+------+---------+----------+----------+------+
| 0 | D2O | 5.5e-06 | 5.98e-06 | 6.4e-06 | True |
| 1 | SMW | 1e-06 | 2.21e-06 | 4.99e-06 | True |
+-------+------+---------+----------+----------+------+
Scalefactors: --------------------------------------------------------------------------------------
+-------+---------------+------+-------+-----+-------+
| index | name | min | value | max | fit |
+-------+---------------+------+-------+-----+-------+
| 0 | Scalefactor 1 | 0.05 | 0.1 | 0.2 | False |
| 1 | Scalefactor 2 | 0.05 | 0.15 | 0.2 | False |
+-------+---------------+------+-------+-----+-------+
Background Parameters: -----------------------------------------------------------------------------
+-------+--------------------------+-------+----------+----------+------+
| index | name | min | value | max | fit |
+-------+--------------------------+-------+----------+----------+------+
| 0 | Background parameter D2O | 5e-10 | 2.23e-06 | 7e-06 | True |
| 1 | Background parameter SMW | 1e-10 | 3.38e-06 | 4.99e-06 | True |
+-------+--------------------------+-------+----------+----------+------+
Backgrounds: ---------------------------------------------------------------------------------------
+-------+----------------+----------+--------------------------+
| index | name | type | source |
+-------+----------------+----------+--------------------------+
| 0 | D2O Background | constant | Background parameter D2O |
| 1 | SMW Background | constant | Background parameter SMW |
+-------+----------------+----------+--------------------------+
Resolution Parameters: -----------------------------------------------------------------------------
+-------+--------------------+------+-------+------+-------+
| index | name | min | value | max | fit |
+-------+--------------------+------+-------+------+-------+
| 0 | Resolution Param 1 | 0.01 | 0.03 | 0.05 | False |
+-------+--------------------+------+-------+------+-------+
Resolutions: ---------------------------------------------------------------------------------------
+-------+--------------+----------+--------------------+
| index | name | type | source |
+-------+--------------+----------+--------------------+
| 0 | Resolution 1 | constant | Resolution Param 1 |
+-------+--------------+----------+--------------------+
Data: ----------------------------------------------------------------------------------------------
+-------+--------------+----------------------+---------------------+---------------------+
| index | name | data | data range | simulation range |
+-------+--------------+----------------------+---------------------+---------------------+
| 0 | Simulation | [] | [] | [0.005, 0.7] |
| 1 | dspc_bil_D2O | Data array: [82 x 3] | [0.011403, 0.59342] | [0.011403, 0.59342] |
| 2 | dspc_bil_smw | Data array: [82 x 3] | [0.011403, 0.59342] | [0.011403, 0.59342] |
+-------+--------------+----------------------+---------------------+---------------------+
Layers: --------------------------------------------------------------------------------------------
+-------+---------------+-------------------------+-------------------+---------------------+-------------------------+--------------+
| index | name | thickness | SLD | roughness | hydration | hydrate with |
+-------+---------------+-------------------------+-------------------+---------------------+-------------------------+--------------+
| 0 | Oxide | Oxide Thickness | Oxide SLD | Substrate Roughness | Oxide Hydration | bulk out |
| 1 | SAM Tails | SAM Tails Thickness | SAM Tails SLD | SAM Roughness | SAM Tails Hydration | bulk out |
| 2 | SAM Heads | SAM Heads Thickness | SAM Heads SLD | SAM Roughness | SAM Heads Hydration | bulk out |
| 3 | Central Water | CW Thickness | CW SLD | Bilayer Roughness | CW Hydration | bulk out |
| 4 | Bilayer Heads | Bilayer Heads Thickness | Bilayer Heads SLD | Bilayer Roughness | Bilayer Heads Hydration | bulk out |
| 5 | Bilayer Tails | Bilayer Tails Thickness | Bilayer Tails SLD | Bilayer Roughness | Bilayer Tails Hydration | bulk out |
+-------+---------------+-------------------------+-------------------+---------------------+-------------------------+--------------+
Contrasts: -----------------------------------------------------------------------------------------
+-------+------+--------------+----------------+-------------------+---------+----------+---------------+--------------+----------+---------------+
| index | name | data | background | background action | bulk in | bulk out | scalefactor | resolution | resample | model |
+-------+------+--------------+----------------+-------------------+---------+----------+---------------+--------------+----------+---------------+
| 0 | D2O | dspc_bil_D2O | D2O Background | add | Silicon | D2O | Scalefactor 1 | Resolution 1 | False | Oxide |
| | | | | | | | | | | SAM Tails |
| | | | | | | | | | | SAM Heads |
| | | | | | | | | | | Central Water |
| | | | | | | | | | | Bilayer Heads |
| | | | | | | | | | | Bilayer Tails |
| | | | | | | | | | | Bilayer Tails |
| | | | | | | | | | | Bilayer Heads |
| 1 | SMW | dspc_bil_smw | SMW Background | add | Silicon | SMW | Scalefactor 2 | Resolution 1 | False | Oxide |
| | | | | | | | | | | SAM Tails |
| | | | | | | | | | | SAM Heads |
| | | | | | | | | | | Central Water |
| | | | | | | | | | | Bilayer Heads |
| | | | | | | | | | | Bilayer Tails |
| | | | | | | | | | | Bilayer Tails |
| | | | | | | | | | | Bilayer Heads |
+-------+------+--------------+----------------+-------------------+---------+----------+---------------+--------------+----------+---------------+
Running the Project#
To run a project in RAT, we first need to define a controls block:
[10]:
controls = RAT.Controls(display='iter')
print(controls)
+------------------+-----------+
| Property | Value |
+------------------+-----------+
| procedure | calculate |
| parallel | single |
| calcSldDuringFit | False |
| resampleMinAngle | 0.9 |
| resampleNPoints | 50 |
| display | iter |
+------------------+-----------+
The default action (“procedure”) is just a single calculation. So call RAT.run() with this, and then plot the results:
[11]:
problem, results = RAT.run(problem, controls)
RAT.plotting.plot_ref_sld(problem, results)
Starting RAT ───────────────────────────────────────────────────────────────────────────────────────────────────────────
Elapsed time is 0.009 seconds
Finished RAT ───────────────────────────────────────────────────────────────────────────────────────────────────────────
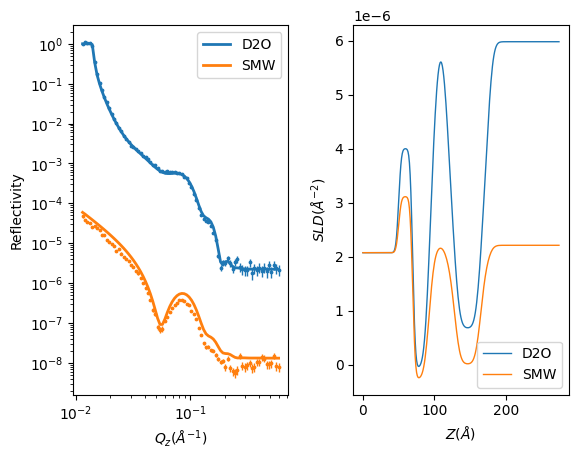